Queue and Stack
Data Structure
- Definition: Computer science에서 효율적인 접근 및 수정을 위해 자료의 조직, 관리, 저장
- 데이터 값의 모임, 데이터 간 관계, 데이터에 적용할 수 있는 함수 및 명령
- 적합한 자료구조 선택을 통해 상대적으로 효율적인 알고리즘 개발 가능
- 구현에 따른 자료구조
- List
- Tuple
- Linked list
- Circular linked list
- Doubly linked list
- Hash table
- 형태에 따른 자료구조
- Linear
- Stack
- Queue
- Deque
- Non-Linear
- Graph
- Tree
- Linear
Queue
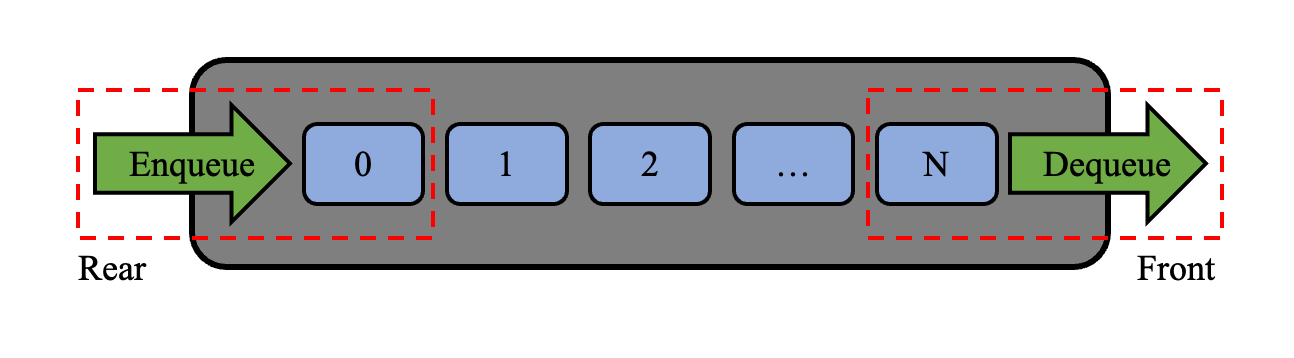
- Method
enQueue
: InsertdeQueue
: Delete
- Application
- 프린터의 인쇄 대기열
- 은행 업무
- 프로세스 관리
- Queue underflow: queue가 비어있을 때
queue.deQueue
를 사용한 경우 발생 - Queue overflow: queue가 가득차있을 때
queue.enQueue
를 사용한 경우 발생
1 | class Queue(): |
Stack
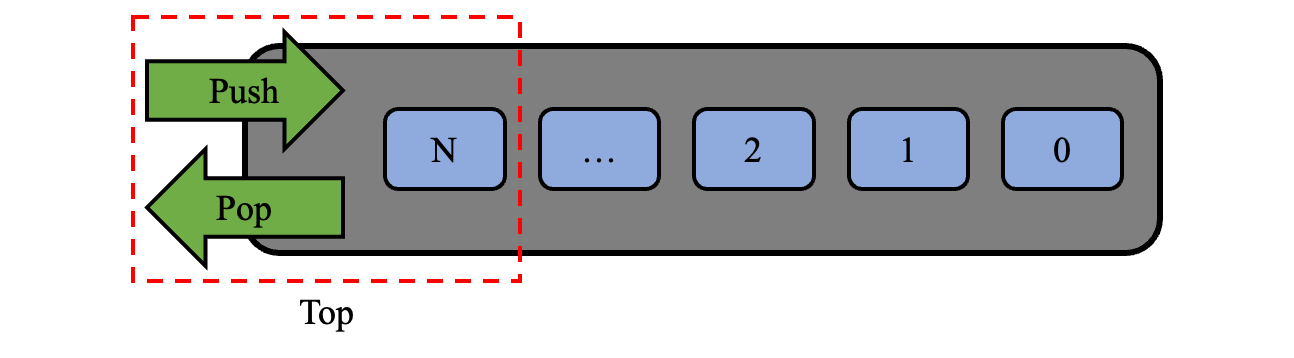
- Method
push
: Insertpop
: Delete
- Application
- Web browser 방문 기록
- 실행 취소 (undo)
- 후위 표기법 계산
- Stack underflow: stack이 비어있을 때
stack.pop
를 사용한 경우 발생 - Stack overflow: stack이 가득차있을 때
stack.push
를 사용한 경우 발생
1 | class Stack(): |
Queue vs. Stack
Queue | Stack | |
---|---|---|
순서 유무 | O | O |
삽입 (insert) | List의 rear | List의 top |
삭제 (delete) | List의 front | List의 top |
구조 (structure) | First-In-First-Out (FIFO) | Last-In-First-Out (LIFO) |